SKlearn模型评估方法
准确率
1.accuracy_score
1 | # 准确率 |
2.metrics
- 宏平均比微平均更合理,但也不是说微平均一无是处,具体使用哪种评测机制,还是要取决于数据集中样本分布
- 宏平均(Macro-averaging),是先对每一个类统计指标值,然后在对所有类求算术平均值。
- 微平均(Micro-averaging),是对数据集中的每一个实例不分类别进行统计建立全局混淆矩阵,然后计算相应指标。
1 | from sklearn import metrics |
- 其中 average 参数有五种:(None, ‘micro’, ‘macro’, ‘weighted’, ‘samples’)
召回率
1 | metrics.recall_score(y_true, y_pred, average='micro') |
F1
1 | metrics.f1_score(y_true, y_pred, average='weighted') |
F2
根据公式计算
1 | from sklearn.metrics import precision_score, recall_score |
混淆矩阵
1 | from sklearn.metrics import confusion_matrix |
分类报告
包含:precision/recall/fi-score/均值/分类个数
1 | # 分类报告:precision/recall/fi-score/均值/分类个数 |
输出
1 | precision recall f1-score support |
kappa score
- kappa score 是一个介于(-1, 1)之间的数. score>0.8 意味着好的分类;0 或更低意味着不好(实际是随机标签)
1 | from sklearn.metrics import cohen_kappa_score |
- ROC 1.计算 ROC 值
1 | import numpy as np |
- 2.ROC 曲线
1 | y = np.array([1, 1, 2, 2]) |
- 海明距离
1 | from sklearn.metrics import hamming_loss |
Jaccard 距离
1 | import numpy as np |
可释方差值(Explained variance score)
1 | from sklearn.metrics import explained_variance_score |
平均绝对误差(Mean absolute error)
1 | from sklearn.metrics import mean_absolute_error |
均方误差(Mean squared error)
1 | from sklearn.metrics import mean_squared_error |
中值绝对误差(Median absolute error)
1 | from sklearn.metrics import median_absolute_error |
R 方值,确定系数
1 | from sklearn.metrics import r2_score |
参考文献
本文作者 : HeoLis
原文链接 : https://ishero.net/SKlearn%E6%A8%A1%E5%9E%8B%E8%AF%84%E4%BC%B0%E6%96%B9%E6%B3%95.html
版权声明 : 本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明出处!
学习、记录、分享、获得
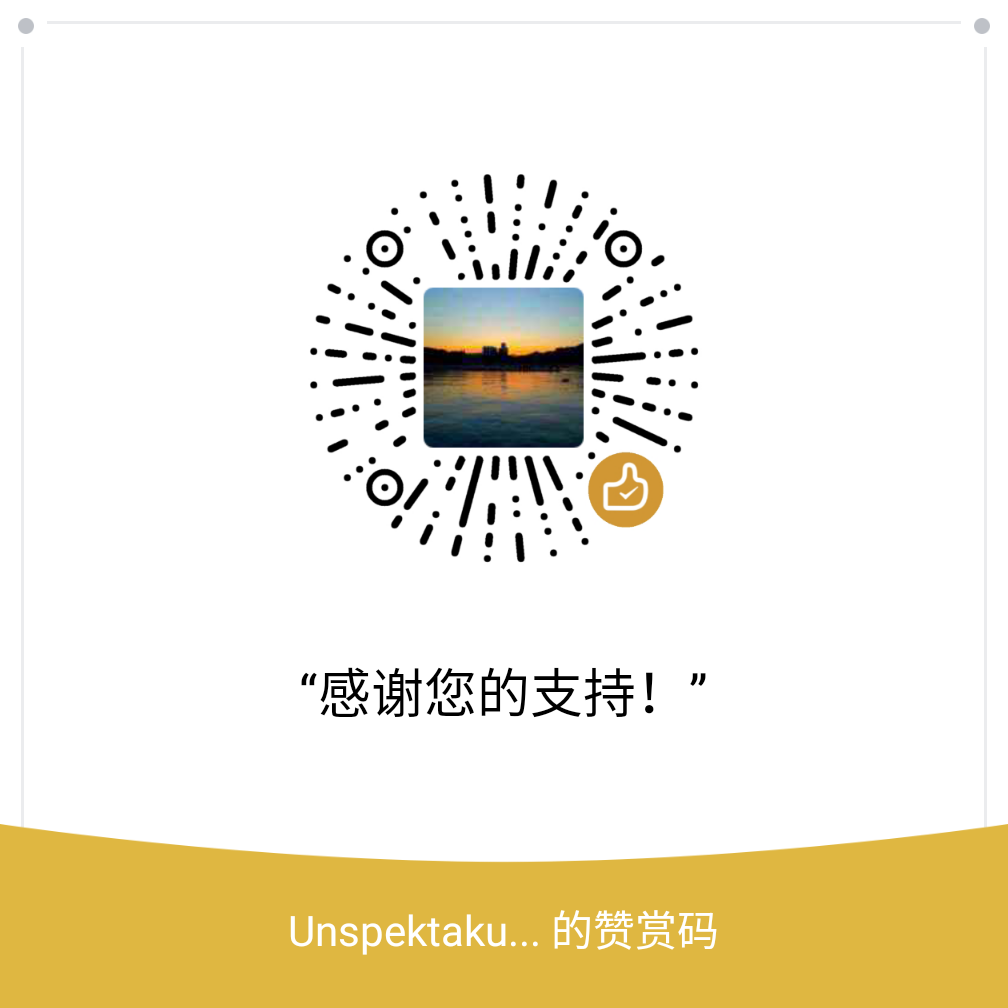